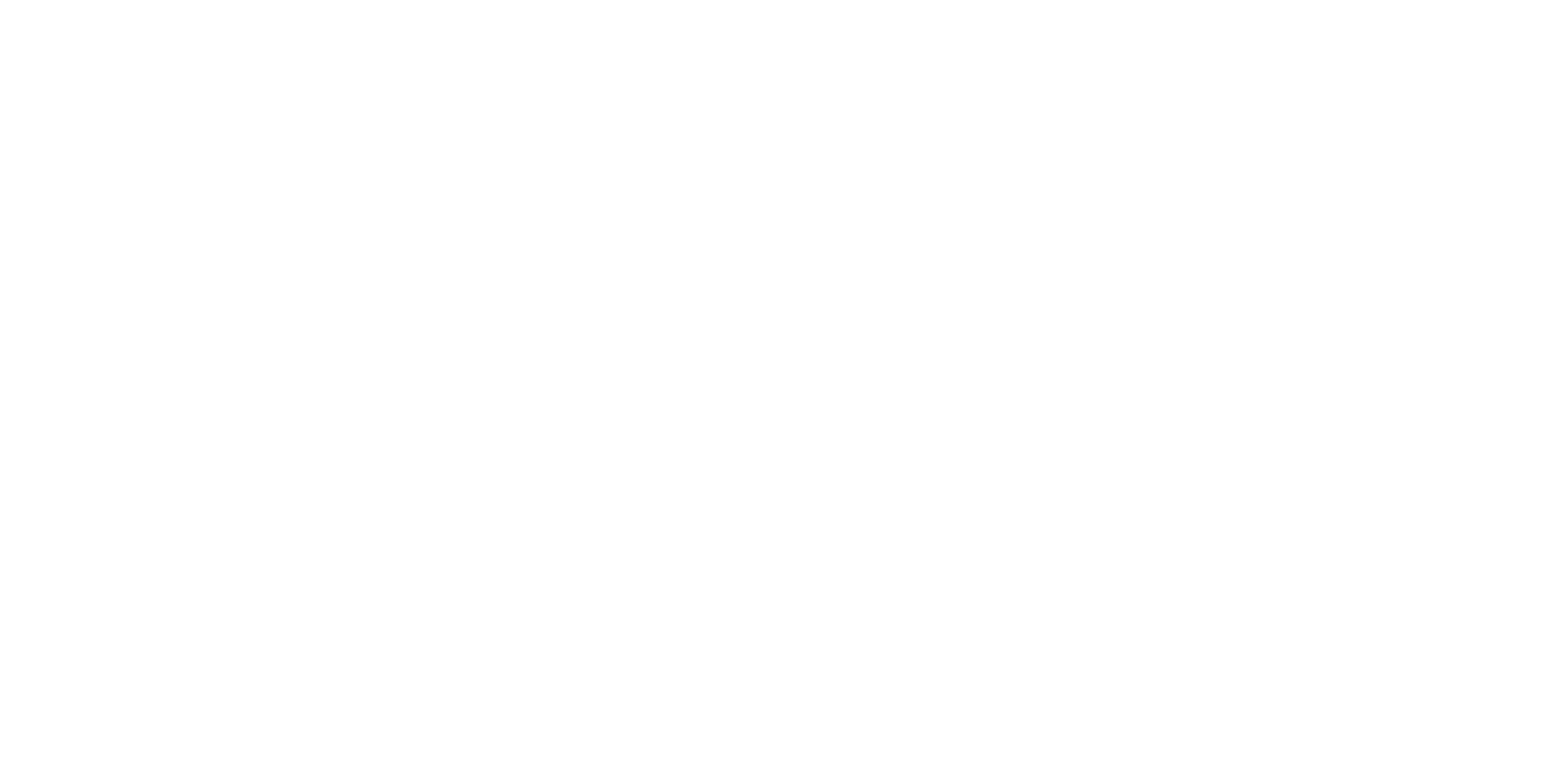
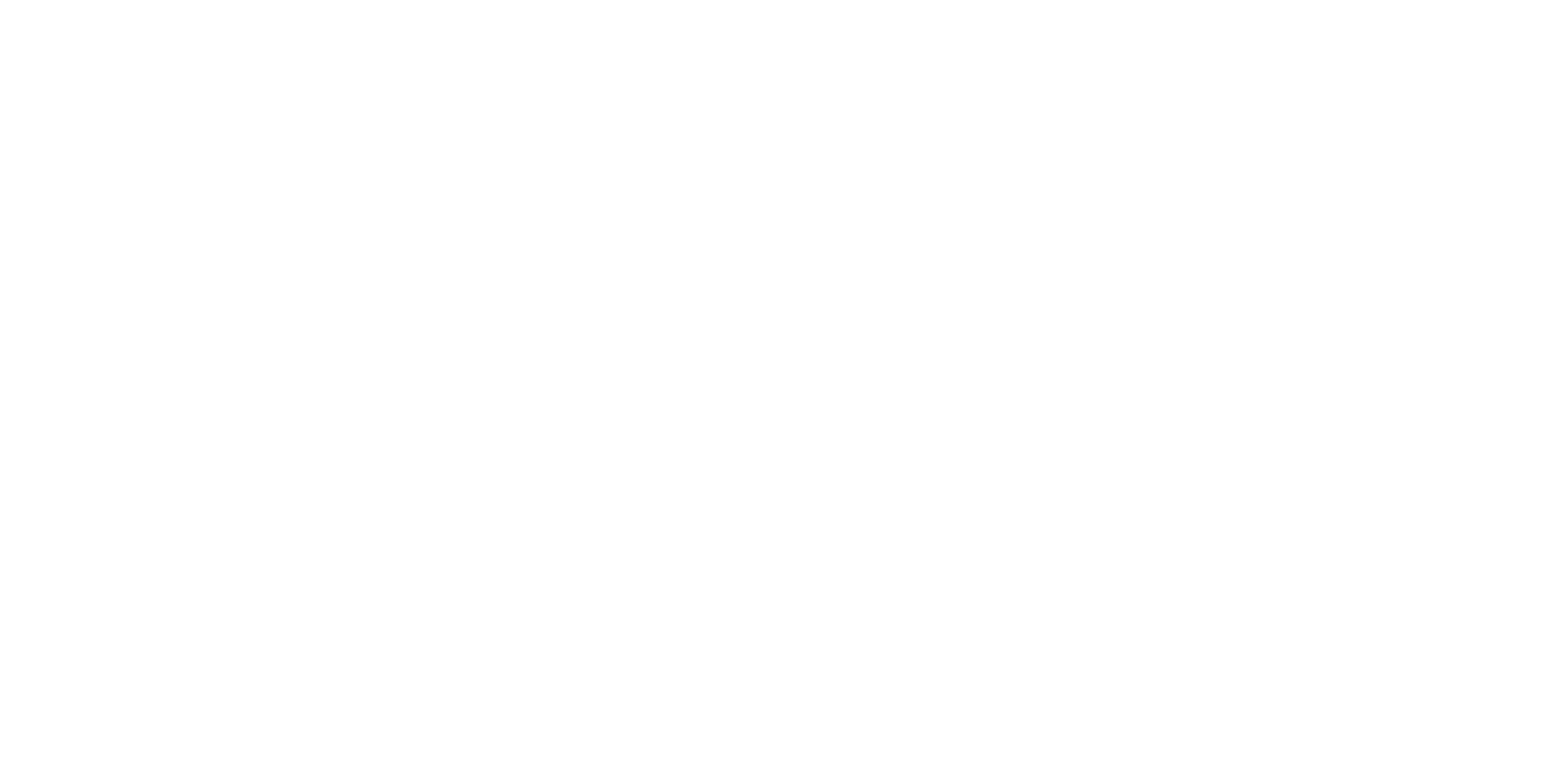



In the evolving landscape of machine learning operations, feature stores have become a crucial component for managing and serving features at scale. This post explores how to implement feature stores using Datazone, offering practical insights for data engineers looking to streamline their ML pipelines.
The Rise of Feature Stores
Feature stores address several key challenges in the ML lifecycle:
Consistency: Ensuring uniform feature definitions across training and inference
Reusability: Enabling feature sharing across different models and teams
Freshness: Managing both batch and real-time feature updates efficiently
Scalability: Handling large-scale feature computation and serving
For data engineers, these capabilities translate to reduced redundancy, improved governance, enhanced operational efficiency, and faster time-to-production for ML models.
Datazone: A Comprehensive Solution for Feature Stores
Datazone provides a robust framework for implementing feature stores. Let's walk through the key components and how they come together to create a powerful feature management system.
Setting Up the Feature Store
First, let's import the necessary components and set up our feature store:
from datazone import (
FeatureStore, entity, feature_group, feature, feature_view, feature_server,
transform, Input, Output, Dataset, Stream, ValueType
)
feature_store = FeatureStore(
name="customer_churn_store",
project="churn_prediction",
offline_store={"type": "dataset", "id": "123123123123"},
online_store={"type": "dataset.alias", "name": "customer_features"},
description="Feature store for customer churn prediction",
tags={"department": "customer_retention", "version": "1.0"}
)
This setup creates a centralized repository for our features, with configurations for both offline (batch) and online (real-time) storage.
Defining Entities
Entities represent the core objects in our domain. Here's how we define a Customer entity:
@entity(feature_store)
class Customer:
name="customer",
primary_keys=["customer_id"],
description="A customer of our service",
value_type=ValueType.STRING
This decorator-based approach provides a clean, declarative way to define entities.
Creating Feature Groups
Feature groups organize related features and their computation logic:
@feature_group(feature_store, Customer)
class CustomerProfile:
name = "customer_profile_features"
description = "Profile features of a customer"
age = feature(ValueType.INT64)
tenure_days = feature(ValueType.INT64)
is_premium = feature(ValueType.INT64)
@transform(
input_mapping={"customer_data": Input(Dataset(id="customer_profile"))}
)
def compute_profile_features(customer_data):
return customer_data.select(
"customer_id",
"age",
"tenure_days",
when(col("subscription_type") == "premium", 1).otherwise(0).alias("is_premium")
)
This feature group includes both feature definitions and the transformation logic to compute them, encapsulating related functionality.
Handling Real-time Features
Datazone supports real-time feature computation, crucial for capturing up-to-date customer behaviors:
@feature_group(feature_store, Customer)
class RealtimeCustomerActivity:
name = "realtime_customer_activity"
description = "Real-time activity features of a customer"
recent_activity_count = feature(ValueType.INT64, realtime=True)
recent_purchase_count = feature(ValueType.INT64, realtime=True)
purchase_ratio = feature(ValueType.FLOAT, realtime=True)
@stream(
input_mapping={"activity_stream": Stream(id="customer_activity_stream")}
)
def process_activity_stream(activity_stream):
return activity_stream.groupBy(
window("timestamp", "5 minutes"),
"customer_id"
).agg(
count("*").alias("recent_activity_count"),
sum(when(col("action") == "purchase", 1).otherwise(0)).alias("recent_purchase_count")
).select(
col("customer_id"),
col("recent_activity_count"),
(col("recent_purchase_count") / col("recent_activity_count")).alias("purchase_ratio")
)
The @stream
decorator defines how to process streaming data, enabling real-time feature updates.
Defining Feature Views
Feature views combine features for specific use cases, such as our churn prediction model:
@feature_view(feature_store)
class ChurnPredictionView:
name = "churn_prediction_features"
entities = [Customer]
features = [
CustomerProfile.age,
CustomerProfile.tenure_days,
CustomerProfile.is_premium,
CustomerBehavior.total_spend,
CustomerBehavior.avg_transaction_value,
CustomerBehavior.transaction_count,
RealtimeCustomerActivity.recent_activity_count,
RealtimeCustomerActivity.recent_purchase_count,
RealtimeCustomerActivity.purchase_ratio
]
ttl = timedelta(minutes=5) # Short TTL for real-time features
This view aggregates both batch and real-time features, providing a comprehensive set of features for our model.
Serving Features
Datazone offers utilities for serving features in both batch and real-time scenarios:
@transform(
input_mapping={
"customer_ids": Input(Dataset(id="customers_to_predict")),
"features": Input(feature_store.get_online_features('churn_prediction_features'))
},
output_mapping={"prediction_input": Output(Dataset(id="churn_prediction_input"))}
)
def prepare_realtime_prediction_input(customer_ids, features):
return customer_ids.join(
features,
on="customer_id",
how="left"
)
@feature_server.endpoint("/predict_churn")
def predict_churn(customer_id: str):
features = feature_store.get_online_features(
feature_view="churn_prediction_features"
)
prediction = churn_model.predict(features)
return {"customer_id": customer_id, "churn_probability": prediction}
These functions demonstrate how to retrieve and use features for both batch processing and real-time predictions.
Conclusion: The Power of Datazone for Feature Stores
Implementing a feature store with Datazone offers several advantages for data engineers:
Unified API: A consistent interface for managing both batch and streaming features
Declarative Definitions: Clear, Python-based feature definitions that enhance readability and maintainability
Scalability: Built on distributed computing frameworks, enabling handling of large-scale data
Flexibility: Support for various data sources and serving patterns to fit diverse use cases
By leveraging Datazone, data engineers can build robust, scalable feature management systems that bridge the gap between data engineering and machine learning. This approach not only accelerates the ML lifecycle but also improves model performance and reliability.
As you implement feature stores in your projects, remember that the key to success lies in thoughtful feature design, consistent management, and seamless integration with your existing data infrastructure. Datazone provides the tools; it's up to us to use them effectively to unlock the full potential of our machine learning initiatives.
#DataEngineering #MachineLearning #FeatureStores #Datazone #MLOps
In the evolving landscape of machine learning operations, feature stores have become a crucial component for managing and serving features at scale. This post explores how to implement feature stores using Datazone, offering practical insights for data engineers looking to streamline their ML pipelines.
The Rise of Feature Stores
Feature stores address several key challenges in the ML lifecycle:
Consistency: Ensuring uniform feature definitions across training and inference
Reusability: Enabling feature sharing across different models and teams
Freshness: Managing both batch and real-time feature updates efficiently
Scalability: Handling large-scale feature computation and serving
For data engineers, these capabilities translate to reduced redundancy, improved governance, enhanced operational efficiency, and faster time-to-production for ML models.
Datazone: A Comprehensive Solution for Feature Stores
Datazone provides a robust framework for implementing feature stores. Let's walk through the key components and how they come together to create a powerful feature management system.
Setting Up the Feature Store
First, let's import the necessary components and set up our feature store:
from datazone import (
FeatureStore, entity, feature_group, feature, feature_view, feature_server,
transform, Input, Output, Dataset, Stream, ValueType
)
feature_store = FeatureStore(
name="customer_churn_store",
project="churn_prediction",
offline_store={"type": "dataset", "id": "123123123123"},
online_store={"type": "dataset.alias", "name": "customer_features"},
description="Feature store for customer churn prediction",
tags={"department": "customer_retention", "version": "1.0"}
)
This setup creates a centralized repository for our features, with configurations for both offline (batch) and online (real-time) storage.
Defining Entities
Entities represent the core objects in our domain. Here's how we define a Customer entity:
@entity(feature_store)
class Customer:
name="customer",
primary_keys=["customer_id"],
description="A customer of our service",
value_type=ValueType.STRING
This decorator-based approach provides a clean, declarative way to define entities.
Creating Feature Groups
Feature groups organize related features and their computation logic:
@feature_group(feature_store, Customer)
class CustomerProfile:
name = "customer_profile_features"
description = "Profile features of a customer"
age = feature(ValueType.INT64)
tenure_days = feature(ValueType.INT64)
is_premium = feature(ValueType.INT64)
@transform(
input_mapping={"customer_data": Input(Dataset(id="customer_profile"))}
)
def compute_profile_features(customer_data):
return customer_data.select(
"customer_id",
"age",
"tenure_days",
when(col("subscription_type") == "premium", 1).otherwise(0).alias("is_premium")
)
This feature group includes both feature definitions and the transformation logic to compute them, encapsulating related functionality.
Handling Real-time Features
Datazone supports real-time feature computation, crucial for capturing up-to-date customer behaviors:
@feature_group(feature_store, Customer)
class RealtimeCustomerActivity:
name = "realtime_customer_activity"
description = "Real-time activity features of a customer"
recent_activity_count = feature(ValueType.INT64, realtime=True)
recent_purchase_count = feature(ValueType.INT64, realtime=True)
purchase_ratio = feature(ValueType.FLOAT, realtime=True)
@stream(
input_mapping={"activity_stream": Stream(id="customer_activity_stream")}
)
def process_activity_stream(activity_stream):
return activity_stream.groupBy(
window("timestamp", "5 minutes"),
"customer_id"
).agg(
count("*").alias("recent_activity_count"),
sum(when(col("action") == "purchase", 1).otherwise(0)).alias("recent_purchase_count")
).select(
col("customer_id"),
col("recent_activity_count"),
(col("recent_purchase_count") / col("recent_activity_count")).alias("purchase_ratio")
)
The @stream
decorator defines how to process streaming data, enabling real-time feature updates.
Defining Feature Views
Feature views combine features for specific use cases, such as our churn prediction model:
@feature_view(feature_store)
class ChurnPredictionView:
name = "churn_prediction_features"
entities = [Customer]
features = [
CustomerProfile.age,
CustomerProfile.tenure_days,
CustomerProfile.is_premium,
CustomerBehavior.total_spend,
CustomerBehavior.avg_transaction_value,
CustomerBehavior.transaction_count,
RealtimeCustomerActivity.recent_activity_count,
RealtimeCustomerActivity.recent_purchase_count,
RealtimeCustomerActivity.purchase_ratio
]
ttl = timedelta(minutes=5) # Short TTL for real-time features
This view aggregates both batch and real-time features, providing a comprehensive set of features for our model.
Serving Features
Datazone offers utilities for serving features in both batch and real-time scenarios:
@transform(
input_mapping={
"customer_ids": Input(Dataset(id="customers_to_predict")),
"features": Input(feature_store.get_online_features('churn_prediction_features'))
},
output_mapping={"prediction_input": Output(Dataset(id="churn_prediction_input"))}
)
def prepare_realtime_prediction_input(customer_ids, features):
return customer_ids.join(
features,
on="customer_id",
how="left"
)
@feature_server.endpoint("/predict_churn")
def predict_churn(customer_id: str):
features = feature_store.get_online_features(
feature_view="churn_prediction_features"
)
prediction = churn_model.predict(features)
return {"customer_id": customer_id, "churn_probability": prediction}
These functions demonstrate how to retrieve and use features for both batch processing and real-time predictions.
Conclusion: The Power of Datazone for Feature Stores
Implementing a feature store with Datazone offers several advantages for data engineers:
Unified API: A consistent interface for managing both batch and streaming features
Declarative Definitions: Clear, Python-based feature definitions that enhance readability and maintainability
Scalability: Built on distributed computing frameworks, enabling handling of large-scale data
Flexibility: Support for various data sources and serving patterns to fit diverse use cases
By leveraging Datazone, data engineers can build robust, scalable feature management systems that bridge the gap between data engineering and machine learning. This approach not only accelerates the ML lifecycle but also improves model performance and reliability.
As you implement feature stores in your projects, remember that the key to success lies in thoughtful feature design, consistent management, and seamless integration with your existing data infrastructure. Datazone provides the tools; it's up to us to use them effectively to unlock the full potential of our machine learning initiatives.
#DataEngineering #MachineLearning #FeatureStores #Datazone #MLOps
In the evolving landscape of machine learning operations, feature stores have become a crucial component for managing and serving features at scale. This post explores how to implement feature stores using Datazone, offering practical insights for data engineers looking to streamline their ML pipelines.
The Rise of Feature Stores
Feature stores address several key challenges in the ML lifecycle:
Consistency: Ensuring uniform feature definitions across training and inference
Reusability: Enabling feature sharing across different models and teams
Freshness: Managing both batch and real-time feature updates efficiently
Scalability: Handling large-scale feature computation and serving
For data engineers, these capabilities translate to reduced redundancy, improved governance, enhanced operational efficiency, and faster time-to-production for ML models.
Datazone: A Comprehensive Solution for Feature Stores
Datazone provides a robust framework for implementing feature stores. Let's walk through the key components and how they come together to create a powerful feature management system.
Setting Up the Feature Store
First, let's import the necessary components and set up our feature store:
from datazone import (
FeatureStore, entity, feature_group, feature, feature_view, feature_server,
transform, Input, Output, Dataset, Stream, ValueType
)
feature_store = FeatureStore(
name="customer_churn_store",
project="churn_prediction",
offline_store={"type": "dataset", "id": "123123123123"},
online_store={"type": "dataset.alias", "name": "customer_features"},
description="Feature store for customer churn prediction",
tags={"department": "customer_retention", "version": "1.0"}
)
This setup creates a centralized repository for our features, with configurations for both offline (batch) and online (real-time) storage.
Defining Entities
Entities represent the core objects in our domain. Here's how we define a Customer entity:
@entity(feature_store)
class Customer:
name="customer",
primary_keys=["customer_id"],
description="A customer of our service",
value_type=ValueType.STRING
This decorator-based approach provides a clean, declarative way to define entities.
Creating Feature Groups
Feature groups organize related features and their computation logic:
@feature_group(feature_store, Customer)
class CustomerProfile:
name = "customer_profile_features"
description = "Profile features of a customer"
age = feature(ValueType.INT64)
tenure_days = feature(ValueType.INT64)
is_premium = feature(ValueType.INT64)
@transform(
input_mapping={"customer_data": Input(Dataset(id="customer_profile"))}
)
def compute_profile_features(customer_data):
return customer_data.select(
"customer_id",
"age",
"tenure_days",
when(col("subscription_type") == "premium", 1).otherwise(0).alias("is_premium")
)
This feature group includes both feature definitions and the transformation logic to compute them, encapsulating related functionality.
Handling Real-time Features
Datazone supports real-time feature computation, crucial for capturing up-to-date customer behaviors:
@feature_group(feature_store, Customer)
class RealtimeCustomerActivity:
name = "realtime_customer_activity"
description = "Real-time activity features of a customer"
recent_activity_count = feature(ValueType.INT64, realtime=True)
recent_purchase_count = feature(ValueType.INT64, realtime=True)
purchase_ratio = feature(ValueType.FLOAT, realtime=True)
@stream(
input_mapping={"activity_stream": Stream(id="customer_activity_stream")}
)
def process_activity_stream(activity_stream):
return activity_stream.groupBy(
window("timestamp", "5 minutes"),
"customer_id"
).agg(
count("*").alias("recent_activity_count"),
sum(when(col("action") == "purchase", 1).otherwise(0)).alias("recent_purchase_count")
).select(
col("customer_id"),
col("recent_activity_count"),
(col("recent_purchase_count") / col("recent_activity_count")).alias("purchase_ratio")
)
The @stream
decorator defines how to process streaming data, enabling real-time feature updates.
Defining Feature Views
Feature views combine features for specific use cases, such as our churn prediction model:
@feature_view(feature_store)
class ChurnPredictionView:
name = "churn_prediction_features"
entities = [Customer]
features = [
CustomerProfile.age,
CustomerProfile.tenure_days,
CustomerProfile.is_premium,
CustomerBehavior.total_spend,
CustomerBehavior.avg_transaction_value,
CustomerBehavior.transaction_count,
RealtimeCustomerActivity.recent_activity_count,
RealtimeCustomerActivity.recent_purchase_count,
RealtimeCustomerActivity.purchase_ratio
]
ttl = timedelta(minutes=5) # Short TTL for real-time features
This view aggregates both batch and real-time features, providing a comprehensive set of features for our model.
Serving Features
Datazone offers utilities for serving features in both batch and real-time scenarios:
@transform(
input_mapping={
"customer_ids": Input(Dataset(id="customers_to_predict")),
"features": Input(feature_store.get_online_features('churn_prediction_features'))
},
output_mapping={"prediction_input": Output(Dataset(id="churn_prediction_input"))}
)
def prepare_realtime_prediction_input(customer_ids, features):
return customer_ids.join(
features,
on="customer_id",
how="left"
)
@feature_server.endpoint("/predict_churn")
def predict_churn(customer_id: str):
features = feature_store.get_online_features(
feature_view="churn_prediction_features"
)
prediction = churn_model.predict(features)
return {"customer_id": customer_id, "churn_probability": prediction}
These functions demonstrate how to retrieve and use features for both batch processing and real-time predictions.
Conclusion: The Power of Datazone for Feature Stores
Implementing a feature store with Datazone offers several advantages for data engineers:
Unified API: A consistent interface for managing both batch and streaming features
Declarative Definitions: Clear, Python-based feature definitions that enhance readability and maintainability
Scalability: Built on distributed computing frameworks, enabling handling of large-scale data
Flexibility: Support for various data sources and serving patterns to fit diverse use cases
By leveraging Datazone, data engineers can build robust, scalable feature management systems that bridge the gap between data engineering and machine learning. This approach not only accelerates the ML lifecycle but also improves model performance and reliability.
As you implement feature stores in your projects, remember that the key to success lies in thoughtful feature design, consistent management, and seamless integration with your existing data infrastructure. Datazone provides the tools; it's up to us to use them effectively to unlock the full potential of our machine learning initiatives.
#DataEngineering #MachineLearning #FeatureStores #Datazone #MLOps
In the evolving landscape of machine learning operations, feature stores have become a crucial component for managing and serving features at scale. This post explores how to implement feature stores using Datazone, offering practical insights for data engineers looking to streamline their ML pipelines.
The Rise of Feature Stores
Feature stores address several key challenges in the ML lifecycle:
Consistency: Ensuring uniform feature definitions across training and inference
Reusability: Enabling feature sharing across different models and teams
Freshness: Managing both batch and real-time feature updates efficiently
Scalability: Handling large-scale feature computation and serving
For data engineers, these capabilities translate to reduced redundancy, improved governance, enhanced operational efficiency, and faster time-to-production for ML models.
Datazone: A Comprehensive Solution for Feature Stores
Datazone provides a robust framework for implementing feature stores. Let's walk through the key components and how they come together to create a powerful feature management system.
Setting Up the Feature Store
First, let's import the necessary components and set up our feature store:
from datazone import (
FeatureStore, entity, feature_group, feature, feature_view, feature_server,
transform, Input, Output, Dataset, Stream, ValueType
)
feature_store = FeatureStore(
name="customer_churn_store",
project="churn_prediction",
offline_store={"type": "dataset", "id": "123123123123"},
online_store={"type": "dataset.alias", "name": "customer_features"},
description="Feature store for customer churn prediction",
tags={"department": "customer_retention", "version": "1.0"}
)
This setup creates a centralized repository for our features, with configurations for both offline (batch) and online (real-time) storage.
Defining Entities
Entities represent the core objects in our domain. Here's how we define a Customer entity:
@entity(feature_store)
class Customer:
name="customer",
primary_keys=["customer_id"],
description="A customer of our service",
value_type=ValueType.STRING
This decorator-based approach provides a clean, declarative way to define entities.
Creating Feature Groups
Feature groups organize related features and their computation logic:
@feature_group(feature_store, Customer)
class CustomerProfile:
name = "customer_profile_features"
description = "Profile features of a customer"
age = feature(ValueType.INT64)
tenure_days = feature(ValueType.INT64)
is_premium = feature(ValueType.INT64)
@transform(
input_mapping={"customer_data": Input(Dataset(id="customer_profile"))}
)
def compute_profile_features(customer_data):
return customer_data.select(
"customer_id",
"age",
"tenure_days",
when(col("subscription_type") == "premium", 1).otherwise(0).alias("is_premium")
)
This feature group includes both feature definitions and the transformation logic to compute them, encapsulating related functionality.
Handling Real-time Features
Datazone supports real-time feature computation, crucial for capturing up-to-date customer behaviors:
@feature_group(feature_store, Customer)
class RealtimeCustomerActivity:
name = "realtime_customer_activity"
description = "Real-time activity features of a customer"
recent_activity_count = feature(ValueType.INT64, realtime=True)
recent_purchase_count = feature(ValueType.INT64, realtime=True)
purchase_ratio = feature(ValueType.FLOAT, realtime=True)
@stream(
input_mapping={"activity_stream": Stream(id="customer_activity_stream")}
)
def process_activity_stream(activity_stream):
return activity_stream.groupBy(
window("timestamp", "5 minutes"),
"customer_id"
).agg(
count("*").alias("recent_activity_count"),
sum(when(col("action") == "purchase", 1).otherwise(0)).alias("recent_purchase_count")
).select(
col("customer_id"),
col("recent_activity_count"),
(col("recent_purchase_count") / col("recent_activity_count")).alias("purchase_ratio")
)
The @stream
decorator defines how to process streaming data, enabling real-time feature updates.
Defining Feature Views
Feature views combine features for specific use cases, such as our churn prediction model:
@feature_view(feature_store)
class ChurnPredictionView:
name = "churn_prediction_features"
entities = [Customer]
features = [
CustomerProfile.age,
CustomerProfile.tenure_days,
CustomerProfile.is_premium,
CustomerBehavior.total_spend,
CustomerBehavior.avg_transaction_value,
CustomerBehavior.transaction_count,
RealtimeCustomerActivity.recent_activity_count,
RealtimeCustomerActivity.recent_purchase_count,
RealtimeCustomerActivity.purchase_ratio
]
ttl = timedelta(minutes=5) # Short TTL for real-time features
This view aggregates both batch and real-time features, providing a comprehensive set of features for our model.
Serving Features
Datazone offers utilities for serving features in both batch and real-time scenarios:
@transform(
input_mapping={
"customer_ids": Input(Dataset(id="customers_to_predict")),
"features": Input(feature_store.get_online_features('churn_prediction_features'))
},
output_mapping={"prediction_input": Output(Dataset(id="churn_prediction_input"))}
)
def prepare_realtime_prediction_input(customer_ids, features):
return customer_ids.join(
features,
on="customer_id",
how="left"
)
@feature_server.endpoint("/predict_churn")
def predict_churn(customer_id: str):
features = feature_store.get_online_features(
feature_view="churn_prediction_features"
)
prediction = churn_model.predict(features)
return {"customer_id": customer_id, "churn_probability": prediction}
These functions demonstrate how to retrieve and use features for both batch processing and real-time predictions.
Conclusion: The Power of Datazone for Feature Stores
Implementing a feature store with Datazone offers several advantages for data engineers:
Unified API: A consistent interface for managing both batch and streaming features
Declarative Definitions: Clear, Python-based feature definitions that enhance readability and maintainability
Scalability: Built on distributed computing frameworks, enabling handling of large-scale data
Flexibility: Support for various data sources and serving patterns to fit diverse use cases
By leveraging Datazone, data engineers can build robust, scalable feature management systems that bridge the gap between data engineering and machine learning. This approach not only accelerates the ML lifecycle but also improves model performance and reliability.
As you implement feature stores in your projects, remember that the key to success lies in thoughtful feature design, consistent management, and seamless integration with your existing data infrastructure. Datazone provides the tools; it's up to us to use them effectively to unlock the full potential of our machine learning initiatives.
#DataEngineering #MachineLearning #FeatureStores #Datazone #MLOps
In the evolving landscape of machine learning operations, feature stores have become a crucial component for managing and serving features at scale. This post explores how to implement feature stores using Datazone, offering practical insights for data engineers looking to streamline their ML pipelines.
The Rise of Feature Stores
Feature stores address several key challenges in the ML lifecycle:
Consistency: Ensuring uniform feature definitions across training and inference
Reusability: Enabling feature sharing across different models and teams
Freshness: Managing both batch and real-time feature updates efficiently
Scalability: Handling large-scale feature computation and serving
For data engineers, these capabilities translate to reduced redundancy, improved governance, enhanced operational efficiency, and faster time-to-production for ML models.
Datazone: A Comprehensive Solution for Feature Stores
Datazone provides a robust framework for implementing feature stores. Let's walk through the key components and how they come together to create a powerful feature management system.
Setting Up the Feature Store
First, let's import the necessary components and set up our feature store:
from datazone import (
FeatureStore, entity, feature_group, feature, feature_view, feature_server,
transform, Input, Output, Dataset, Stream, ValueType
)
feature_store = FeatureStore(
name="customer_churn_store",
project="churn_prediction",
offline_store={"type": "dataset", "id": "123123123123"},
online_store={"type": "dataset.alias", "name": "customer_features"},
description="Feature store for customer churn prediction",
tags={"department": "customer_retention", "version": "1.0"}
)
This setup creates a centralized repository for our features, with configurations for both offline (batch) and online (real-time) storage.
Defining Entities
Entities represent the core objects in our domain. Here's how we define a Customer entity:
@entity(feature_store)
class Customer:
name="customer",
primary_keys=["customer_id"],
description="A customer of our service",
value_type=ValueType.STRING
This decorator-based approach provides a clean, declarative way to define entities.
Creating Feature Groups
Feature groups organize related features and their computation logic:
@feature_group(feature_store, Customer)
class CustomerProfile:
name = "customer_profile_features"
description = "Profile features of a customer"
age = feature(ValueType.INT64)
tenure_days = feature(ValueType.INT64)
is_premium = feature(ValueType.INT64)
@transform(
input_mapping={"customer_data": Input(Dataset(id="customer_profile"))}
)
def compute_profile_features(customer_data):
return customer_data.select(
"customer_id",
"age",
"tenure_days",
when(col("subscription_type") == "premium", 1).otherwise(0).alias("is_premium")
)
This feature group includes both feature definitions and the transformation logic to compute them, encapsulating related functionality.
Handling Real-time Features
Datazone supports real-time feature computation, crucial for capturing up-to-date customer behaviors:
@feature_group(feature_store, Customer)
class RealtimeCustomerActivity:
name = "realtime_customer_activity"
description = "Real-time activity features of a customer"
recent_activity_count = feature(ValueType.INT64, realtime=True)
recent_purchase_count = feature(ValueType.INT64, realtime=True)
purchase_ratio = feature(ValueType.FLOAT, realtime=True)
@stream(
input_mapping={"activity_stream": Stream(id="customer_activity_stream")}
)
def process_activity_stream(activity_stream):
return activity_stream.groupBy(
window("timestamp", "5 minutes"),
"customer_id"
).agg(
count("*").alias("recent_activity_count"),
sum(when(col("action") == "purchase", 1).otherwise(0)).alias("recent_purchase_count")
).select(
col("customer_id"),
col("recent_activity_count"),
(col("recent_purchase_count") / col("recent_activity_count")).alias("purchase_ratio")
)
The @stream
decorator defines how to process streaming data, enabling real-time feature updates.
Defining Feature Views
Feature views combine features for specific use cases, such as our churn prediction model:
@feature_view(feature_store)
class ChurnPredictionView:
name = "churn_prediction_features"
entities = [Customer]
features = [
CustomerProfile.age,
CustomerProfile.tenure_days,
CustomerProfile.is_premium,
CustomerBehavior.total_spend,
CustomerBehavior.avg_transaction_value,
CustomerBehavior.transaction_count,
RealtimeCustomerActivity.recent_activity_count,
RealtimeCustomerActivity.recent_purchase_count,
RealtimeCustomerActivity.purchase_ratio
]
ttl = timedelta(minutes=5) # Short TTL for real-time features
This view aggregates both batch and real-time features, providing a comprehensive set of features for our model.
Serving Features
Datazone offers utilities for serving features in both batch and real-time scenarios:
@transform(
input_mapping={
"customer_ids": Input(Dataset(id="customers_to_predict")),
"features": Input(feature_store.get_online_features('churn_prediction_features'))
},
output_mapping={"prediction_input": Output(Dataset(id="churn_prediction_input"))}
)
def prepare_realtime_prediction_input(customer_ids, features):
return customer_ids.join(
features,
on="customer_id",
how="left"
)
@feature_server.endpoint("/predict_churn")
def predict_churn(customer_id: str):
features = feature_store.get_online_features(
feature_view="churn_prediction_features"
)
prediction = churn_model.predict(features)
return {"customer_id": customer_id, "churn_probability": prediction}
These functions demonstrate how to retrieve and use features for both batch processing and real-time predictions.
Conclusion: The Power of Datazone for Feature Stores
Implementing a feature store with Datazone offers several advantages for data engineers:
Unified API: A consistent interface for managing both batch and streaming features
Declarative Definitions: Clear, Python-based feature definitions that enhance readability and maintainability
Scalability: Built on distributed computing frameworks, enabling handling of large-scale data
Flexibility: Support for various data sources and serving patterns to fit diverse use cases
By leveraging Datazone, data engineers can build robust, scalable feature management systems that bridge the gap between data engineering and machine learning. This approach not only accelerates the ML lifecycle but also improves model performance and reliability.
As you implement feature stores in your projects, remember that the key to success lies in thoughtful feature design, consistent management, and seamless integration with your existing data infrastructure. Datazone provides the tools; it's up to us to use them effectively to unlock the full potential of our machine learning initiatives.
#DataEngineering #MachineLearning #FeatureStores #Datazone #MLOps
In the evolving landscape of machine learning operations, feature stores have become a crucial component for managing and serving features at scale. This post explores how to implement feature stores using Datazone, offering practical insights for data engineers looking to streamline their ML pipelines.
The Rise of Feature Stores
Feature stores address several key challenges in the ML lifecycle:
Consistency: Ensuring uniform feature definitions across training and inference
Reusability: Enabling feature sharing across different models and teams
Freshness: Managing both batch and real-time feature updates efficiently
Scalability: Handling large-scale feature computation and serving
For data engineers, these capabilities translate to reduced redundancy, improved governance, enhanced operational efficiency, and faster time-to-production for ML models.
Datazone: A Comprehensive Solution for Feature Stores
Datazone provides a robust framework for implementing feature stores. Let's walk through the key components and how they come together to create a powerful feature management system.
Setting Up the Feature Store
First, let's import the necessary components and set up our feature store:
from datazone import (
FeatureStore, entity, feature_group, feature, feature_view, feature_server,
transform, Input, Output, Dataset, Stream, ValueType
)
feature_store = FeatureStore(
name="customer_churn_store",
project="churn_prediction",
offline_store={"type": "dataset", "id": "123123123123"},
online_store={"type": "dataset.alias", "name": "customer_features"},
description="Feature store for customer churn prediction",
tags={"department": "customer_retention", "version": "1.0"}
)
This setup creates a centralized repository for our features, with configurations for both offline (batch) and online (real-time) storage.
Defining Entities
Entities represent the core objects in our domain. Here's how we define a Customer entity:
@entity(feature_store)
class Customer:
name="customer",
primary_keys=["customer_id"],
description="A customer of our service",
value_type=ValueType.STRING
This decorator-based approach provides a clean, declarative way to define entities.
Creating Feature Groups
Feature groups organize related features and their computation logic:
@feature_group(feature_store, Customer)
class CustomerProfile:
name = "customer_profile_features"
description = "Profile features of a customer"
age = feature(ValueType.INT64)
tenure_days = feature(ValueType.INT64)
is_premium = feature(ValueType.INT64)
@transform(
input_mapping={"customer_data": Input(Dataset(id="customer_profile"))}
)
def compute_profile_features(customer_data):
return customer_data.select(
"customer_id",
"age",
"tenure_days",
when(col("subscription_type") == "premium", 1).otherwise(0).alias("is_premium")
)
This feature group includes both feature definitions and the transformation logic to compute them, encapsulating related functionality.
Handling Real-time Features
Datazone supports real-time feature computation, crucial for capturing up-to-date customer behaviors:
@feature_group(feature_store, Customer)
class RealtimeCustomerActivity:
name = "realtime_customer_activity"
description = "Real-time activity features of a customer"
recent_activity_count = feature(ValueType.INT64, realtime=True)
recent_purchase_count = feature(ValueType.INT64, realtime=True)
purchase_ratio = feature(ValueType.FLOAT, realtime=True)
@stream(
input_mapping={"activity_stream": Stream(id="customer_activity_stream")}
)
def process_activity_stream(activity_stream):
return activity_stream.groupBy(
window("timestamp", "5 minutes"),
"customer_id"
).agg(
count("*").alias("recent_activity_count"),
sum(when(col("action") == "purchase", 1).otherwise(0)).alias("recent_purchase_count")
).select(
col("customer_id"),
col("recent_activity_count"),
(col("recent_purchase_count") / col("recent_activity_count")).alias("purchase_ratio")
)
The @stream
decorator defines how to process streaming data, enabling real-time feature updates.
Defining Feature Views
Feature views combine features for specific use cases, such as our churn prediction model:
@feature_view(feature_store)
class ChurnPredictionView:
name = "churn_prediction_features"
entities = [Customer]
features = [
CustomerProfile.age,
CustomerProfile.tenure_days,
CustomerProfile.is_premium,
CustomerBehavior.total_spend,
CustomerBehavior.avg_transaction_value,
CustomerBehavior.transaction_count,
RealtimeCustomerActivity.recent_activity_count,
RealtimeCustomerActivity.recent_purchase_count,
RealtimeCustomerActivity.purchase_ratio
]
ttl = timedelta(minutes=5) # Short TTL for real-time features
This view aggregates both batch and real-time features, providing a comprehensive set of features for our model.
Serving Features
Datazone offers utilities for serving features in both batch and real-time scenarios:
@transform(
input_mapping={
"customer_ids": Input(Dataset(id="customers_to_predict")),
"features": Input(feature_store.get_online_features('churn_prediction_features'))
},
output_mapping={"prediction_input": Output(Dataset(id="churn_prediction_input"))}
)
def prepare_realtime_prediction_input(customer_ids, features):
return customer_ids.join(
features,
on="customer_id",
how="left"
)
@feature_server.endpoint("/predict_churn")
def predict_churn(customer_id: str):
features = feature_store.get_online_features(
feature_view="churn_prediction_features"
)
prediction = churn_model.predict(features)
return {"customer_id": customer_id, "churn_probability": prediction}
These functions demonstrate how to retrieve and use features for both batch processing and real-time predictions.
Conclusion: The Power of Datazone for Feature Stores
Implementing a feature store with Datazone offers several advantages for data engineers:
Unified API: A consistent interface for managing both batch and streaming features
Declarative Definitions: Clear, Python-based feature definitions that enhance readability and maintainability
Scalability: Built on distributed computing frameworks, enabling handling of large-scale data
Flexibility: Support for various data sources and serving patterns to fit diverse use cases
By leveraging Datazone, data engineers can build robust, scalable feature management systems that bridge the gap between data engineering and machine learning. This approach not only accelerates the ML lifecycle but also improves model performance and reliability.
As you implement feature stores in your projects, remember that the key to success lies in thoughtful feature design, consistent management, and seamless integration with your existing data infrastructure. Datazone provides the tools; it's up to us to use them effectively to unlock the full potential of our machine learning initiatives.
#DataEngineering #MachineLearning #FeatureStores #Datazone #MLOps